Goの基本的なテスト方法
|
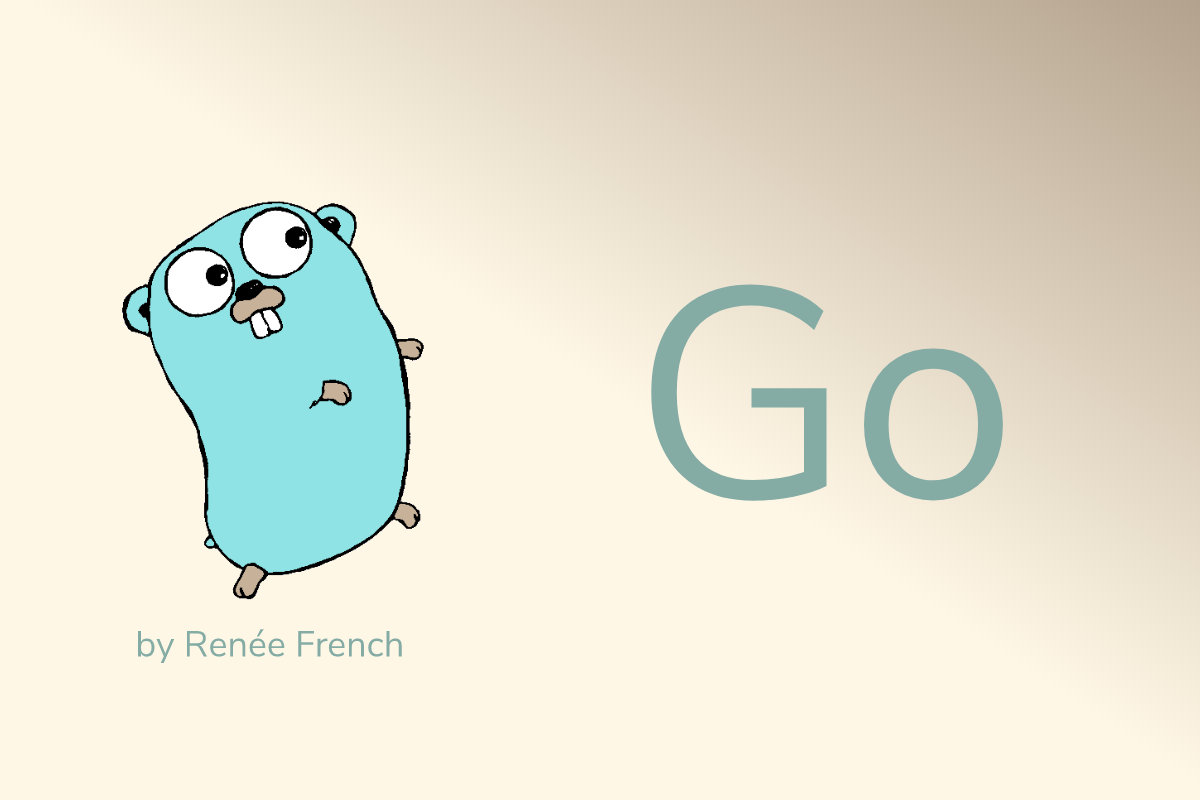
Goの基本的なテスト方法について整理しました。Goには標準でテスト機能が備わっており、関数ごとにテストを実行したり、網羅率をチェックできて便利です。
テストコード実装
app
├── main.go
└── main_test.go
メインの処理が書かれた main.go
に対し、テストが書かれたファイルを main_test.go
という名前にします。
hogehoge.go
というファイルの場合は hogehoge_test.go
となります。
今回はそれぞれ次のような中身でテストしました。
main.go
package main
import (
"fmt"
)
func Calculate(x int) (result int) {
result = x + 2
return result
}
func main() {
result := Calculate(3)
fmt.Printf("3 + 2 = %!v(MISSING)\n", result)
}
main_test.go
package main
import (
"testing"
)
// シンプルなテストコード
func TestCalculate(t *testing.T) {
output := Calculate(2)
if output != 4 {
t.Errorf("Calculate(2) = %!v(MISSING), expected 4", output)
}
}
// テーブル駆動テストの手法に沿ったコード
func TestTableCalculate(t *testing.T) {
var tests = []struct {
input int
expected int
}{
{2, 4},
{-1, 1},
{0, 2},
{-5, -3},
{999, 1001},
}
for _, test := range tests {
output := Calculate(test.input)
if output != test.expected {
t.Errorf("Test Failed: input %!v(MISSING), got %!v(MISSING), want %!v(MISSING)", test.input, output, test.expected)
}
}
}
TestTableCalculate
で実装しているテストコードは、テーブル駆動テストという手法にのっとっています。いくつかのテストケースを網羅したい時に便利です。ここでは「負から正」になる場合、「桁が繰り上がる」場合、などのケースを網羅しています。
参考:TableDrivenTests
参考:An Introduction to Testing in Go
go test コマンド
go test
コマンドで、気軽にテストを実行できます。
# テストを実行
go test
# 各テストの実行と処理時間もチェック
go test -v
# テストカバレッジ(網羅率)もチェック
go test -cover
# テスト関数を指定して実行
go test -run "TestHogehoge" ./package_name
特に -cover
オプションでの網羅率チェックが非常に便利です
# go test -coverの実行結果
PASS
coverage: 50.0%!o(MISSING)f statements
ok github.com/hodanov/go_test 0.005s
網羅率のチェックについて
網羅率についてもう少し見てみます。
先のコマンドでは、網羅率のみチェックしていますが、どの機能をパスしたのか、どの機能がテストされていないのかがわかりません。
それを調べるには、次のように一度カバープロファイルを出力し、 go tool cover
コマンドを実行します。
# カバープロファイルを出力
go test -coverprofile=c.out
# 実装した各functionについて網羅率をチェック
go tool cover -func=c.out
# ↓ go tool cover -func=c.outの出力結果例
github.com/hodanov/go_test/main.go:7: Calculate 100.0%!
(MISSING)github.com/hodanov/go_test/main.go:12: main 0.0%!
(MISSING)total: (statements) 50.0%!
(MISSING)```
## まとめ
Goの基本的なテスト方法について整理しました。これまではまともにテストを書いてこなかったのですが、これからは積極的にテストコードも書いていく所存です。